Build and Deploy a Full-Stack MongoDB, Express, React, Node.js App: A Comprehensive Guide

Are you looking to build a modern, full-stack web application? If so, then you'll need to master the MERN stack: MongoDB, Express, React, and Node.js. This powerful combination of technologies will allow you to create dynamic, interactive web applications that can handle complex data and user interactions.
4.4 out of 5
Language | : | English |
File size | : | 11338 KB |
Text-to-Speech | : | Enabled |
Screen Reader | : | Supported |
Enhanced typesetting | : | Enabled |
Print length | : | 161 pages |
Lending | : | Enabled |
In this comprehensive guide, we'll walk you through the entire process of building and deploying a full-stack MERN application. We'll start by setting up the necessary tools and environment, and then we'll dive into building the application itself. We'll cover everything from creating the database schema to writing the React components and Node.js controllers. Finally, we'll show you how to deploy your application to a live server.
What is the MERN Stack?
The MERN stack is a combination of four powerful technologies: MongoDB, Express, React, and Node.js. Each of these technologies plays a specific role in the development of a full-stack web application:
- MongoDB is a NoSQL database that is designed to store and manage large amounts of data. It is a popular choice for web applications because it is scalable, flexible, and easy to use.
- Express is a web framework for Node.js that makes it easy to create fast, scalable web applications. It provides a variety of features out of the box, such as routing, middleware, and template engines.
- React is a JavaScript library for building user interfaces. It is known for its declarative programming style and its focus on performance. React is used to create interactive, dynamic web applications.
- Node.js is a JavaScript runtime environment that allows you to run JavaScript code on the server side. It is a popular choice for building web applications because it is fast, efficient, and easy to use.
Why Use the MERN Stack?
There are many benefits to using the MERN stack for web development:
- Full-stack: The MERN stack provides a complete set of technologies for building full-stack web applications. This means that you can use the same technologies to build both the front-end and back-end of your application.
- Scalable: The MERN stack is designed to scale to meet the demands of large, complex web applications. MongoDB is a scalable database that can handle large amounts of data, and Express and Node.js are both designed to handle high traffic volumes.
- Flexible: The MERN stack is a flexible framework that allows you to customize your application to meet your specific needs. You can choose which technologies to use for each part of your application, and you can easily integrate third-party libraries and services.
- Easy to use: The MERN stack is a relatively easy-to-use framework for web development. MongoDB has a simple data model, Express is a lightweight framework, and React is a declarative programming language. This makes it easy to get started with the MERN stack, even if you are a beginner web developer.
Getting Started with the MERN Stack
To get started with the MERN stack, you will need to install the following software:
- Node.js
- MongoDB
- Express
- React
You can install these packages using the following commands:
bash npm install -g nodejs npm install -g mongodb npm install -g express npm install -g react
Once you have installed the necessary software, you can create a new MERN application by running the following command:
bash mkdir my-mern-app cd my-mern-app npm init -y
This command will create a new directory for your application and initialize a new npm project. You can now add the MERN stack dependencies to your project by running the following command:
bash npm install --save express mongodb react react-dom
This command will install the Express, MongoDB, React, and React DOM packages to your project. You can now create a new Express application by running the following command:
bash touch server.js
Add the following code to your server.js file:
javascript const express = require('express'); const app = express(); const port = 3000;
app.get('/', (req, res) => { res.send('Hello, world!'); });
app.listen(port, () => { console.log(`Example app listening on port ${port}!`); });
This code creates a new Express application and listens on port 3000. You can now start your Express application by running the following command:
bash node server.js
This command will start your Express application and open a new terminal window. You should now see the following output in your terminal window:
bash Example app listening on port 3000!
You can now visit http://localhost:3000 in your browser to see your Express application in action. You should see the following message in your browser:
Hello, world!
Building a Full-Stack MERN Application
Now that you have a basic understanding of the MERN stack, let's build a full-stack web application. We'll start by creating a new MongoDB database and schema.
bash mongo use my-mern-app db.createCollection('users') db.users.insert({ name: 'John Doe', email: '[email protected]' })
This command will create a new MongoDB database named my-mern-app and a new collection named users. The collection will contain a single document with the name John Doe and the email address [email protected].
Now that we have a database and schema, let's create a new React component to display a list of users. We'll call this component UserList.js:
javascript import React,{useState, useEffect }from 'react'; import axios from 'axios';
const UserList = () => { const [users, setUsers] = useState([]);
useEffect(() => { axios.get('http://localhost:3000/api/users') .then(res => { setUsers(res.data); }) .catch(err => { console.log(err); }); }, []);
return (
- {users.map(user => (
- {user.name}
))}
); };
export default UserList;
This component uses the useState and useEffect hooks to fetch a list of users from the API and display them in a list. The component also uses axios to make the API request.
Now that we have a React component to display a list of users, let's create a Node.js controller to handle the API request. We'll call this controller UserController.js:
javascript const express = require('express'); const router = express.Router(); const User = require('../models/User');
router.get('/api/users', async (req, res) => { try { const users = await User.find(); res.json(users); }catch (err){res.json({ error: err }); }});
module.exports = router;
This controller uses the Express router to handle the API request. The controller also uses the User model to find and return a list of users.
Now that we have a React component and a Node.js controller, let's put them all together to create a full-stack MERN application. We'll start by creating a new Express application:
bash touch app.js
Add the following code to your app.js file:
javascript const express = require('express'); const app = express(); const port = 3000; const UserController = require('./controllers/UserController');
app.use(express.json()); app.use('/api/users', UserController);
app.listen(port, () =>
4.4 out of 5
Language | : | English |
File size | : | 11338 KB |
Text-to-Speech | : | Enabled |
Screen Reader | : | Supported |
Enhanced typesetting | : | Enabled |
Print length | : | 161 pages |
Lending | : | Enabled |
Do you want to contribute by writing guest posts on this blog?
Please contact us and send us a resume of previous articles that you have written.
Book
Novel
Page
Chapter
Text
Story
Genre
Reader
Library
Paperback
E-book
Magazine
Newspaper
Paragraph
Sentence
Bookmark
Shelf
Glossary
Bibliography
Foreword
Preface
Synopsis
Annotation
Footnote
Manuscript
Scroll
Codex
Tome
Bestseller
Classics
Library card
Narrative
Biography
Autobiography
Memoir
Reference
Encyclopedia
Matt Titus
Knowledge Flow
Glenn Rudin
Scott Sumner
Gordon H Chang
Greg Boyd
Stalin Kay
Glenn Dakin
Steve Caplin
Mark Zwonitzer
Grace M Cho
Patti Delano
John Hall
Tony Cleaver
Lisa Gache
John Sefton
Sam Tranum
Hans Keilson
Margaret Visser
Greg W Prince
Light bulbAdvertise smarter! Our strategic ad space ensures maximum exposure. Reserve your spot today!
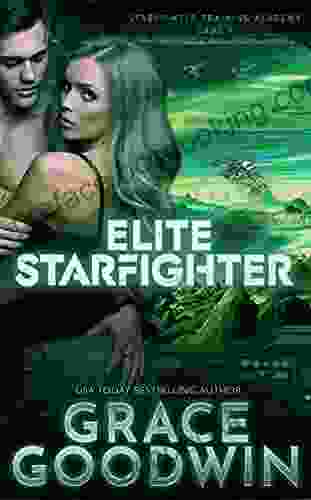

- Giovanni MitchellFollow ·15.5k
- Herbert CoxFollow ·9.4k
- Gary ReedFollow ·15.3k
- Bob CooperFollow ·13.9k
- Thomas MannFollow ·4.2k
- W. Somerset MaughamFollow ·11.4k
- Jimmy ButlerFollow ·5.2k
- Tyrone PowellFollow ·4k
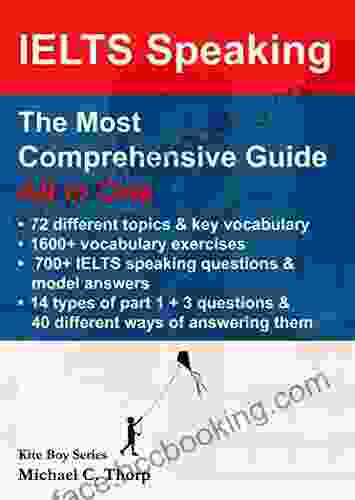

Master IELTS Speaking: The Ultimate Guide to Success
Kickstart Your IELTS...
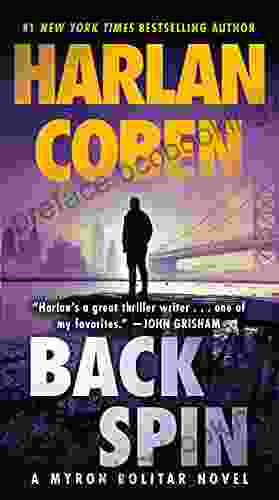

Back Spin: A Thrilling Myron Bolitar Novel
Get ready to embark on a...
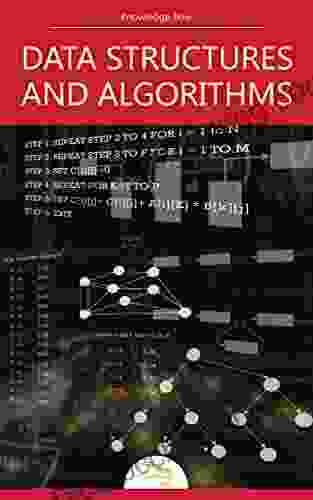

Data Structures and Algorithms: A Comprehensive Guide to...
In the ever-evolving...
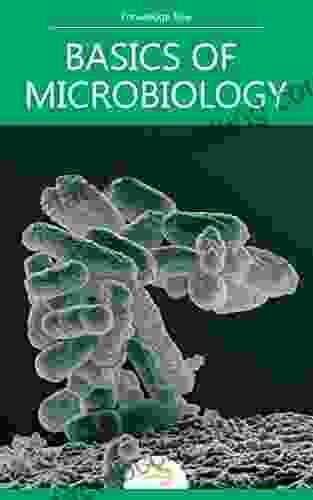

Unveiling the Basics of Microbiology: A Comprehensive...
The world of...
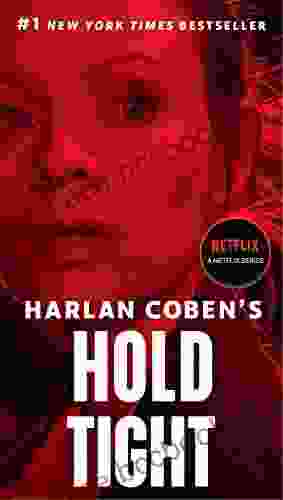

Hold Tight Suspense Thriller: A Gripping Page-Turner That...
Are you ready for a suspense thriller that...
4.4 out of 5
Language | : | English |
File size | : | 11338 KB |
Text-to-Speech | : | Enabled |
Screen Reader | : | Supported |
Enhanced typesetting | : | Enabled |
Print length | : | 161 pages |
Lending | : | Enabled |